Lab 9 Mapping
The goal of this lab was to use sensor data and transformation matrices in order to create a map of the in-lab arena..
Gathering Data
In order to collect 360 degree data, I used orientation control to incrementally rotate by robot in a full circle in 10 degree segments. I limited each turn to 500 ms in order to avoid too much oscillation due to the integral and derivative control aspects. After the turn was completed, the robot would stay in place and collect 4 data points for each TOF sensor. Because my TOF sensors are 180 degrees apart (one on front and one on the back of the bot) this gave me two datasets from which to estimate the map and get an average reading from. Each TOF would provide a mirrored version of that location relative to the other. Similar to past labs, I used boolean flags to control the mapping process, however I could have done it all within the switch case.
A big challenge was making sure my robot actually spun on axis to get as accurate a set of readings as possible. I managed this in two ways: (1) making the robot spin pretty slowly and (2) adding a "calibration" constant of sort to my turning function, so that the right side of motors were always powered with a PWM that was 5 less than the left motors. The code I used is shown below, plus a video of the robot collecting data...
if(MAP_ON){ | |
for (int i = 0; i < 360; i = i + 10) | |
{ | |
float ref = i; | |
Serial.println(ref); | |
startTime = millis(); | |
while ((millis() - startTime) < 500) | |
{ | |
currTime = (float) millis(); | |
dt_ang = (currTime-lastTime)/1000.0; | |
lastTime = currTime; | |
if (!myICM.dataReady()) | |
continue; | |
myICM.getAGMT(); | |
yaw = yaw + myICM.gyrZ()*dt_ang; | |
//int y = (int) yaw % 360; | |
//yaw = (float) y; | |
//Serial.print(yaw); | |
//Serial.println(" | "); | |
pwm = ang_pid(yaw, ref); | |
if(pwm > 0) | |
turn(-1, pwm); | |
else | |
turn(1, -pwm); | |
pwm_ang_data[pwm_counter] = pwm; | |
pwm_counter++; | |
//Serial.println(pwm); | |
} | |
stop(); | |
//after angle is achieved | |
int k = 0; | |
float TOF1; float TOF2; | |
while (k <= 3) { | |
stop(); | |
Serial.println("recording data"); | |
if (distanceSensor1.checkForDataReady()) { | |
TOF1 = distanceSensor1.getDistance(); | |
distanceSensor1.clearInterrupt(); | |
distanceSensor1.stopRanging(); | |
distanceSensor1.startRanging(); | |
} | |
if (distanceSensor2.checkForDataReady()) { | |
TOF2 = distanceSensor2.getDistance(); | |
distanceSensor2.clearInterrupt(); | |
distanceSensor2.stopRanging(); | |
distanceSensor2.startRanging(); | |
} | |
time_data[count] = currTime; | |
distance1_data[count] = TOF1; | |
distance2_data[count] = TOF2; | |
gyro_yaw[count] = yaw; | |
k++; | |
count++; | |
} | |
} | |
stop(); | |
MAP_ON = false; | |
} |
Preliminary Plotting + Transformation Matrices
I placed the robot at each spot in the arena and ran my mapping command at each location. I then plotted the TOF data in a polar plot against the yaw data (in radians! I first did degrees and it looked crazy...). From this, I could already see the shape of the room starting to come together.
 1.png)
 1.png)
 1.png)
.png)
After collecting the data, I began working on transformation matrices to be able to go from polar to cartesian coordinates. I applied the following to each data point: x = distance * cos(theta) and y = distance * sin(theta). From there, I offset the values by the coordinate from which that data point was taken (for example, (-3, -2) had me subtracting 3*304.8mm from x and 2*304.8mm from y). Some transformations also had different signs as needed, to get the correct orientation. The code for that is shown below.
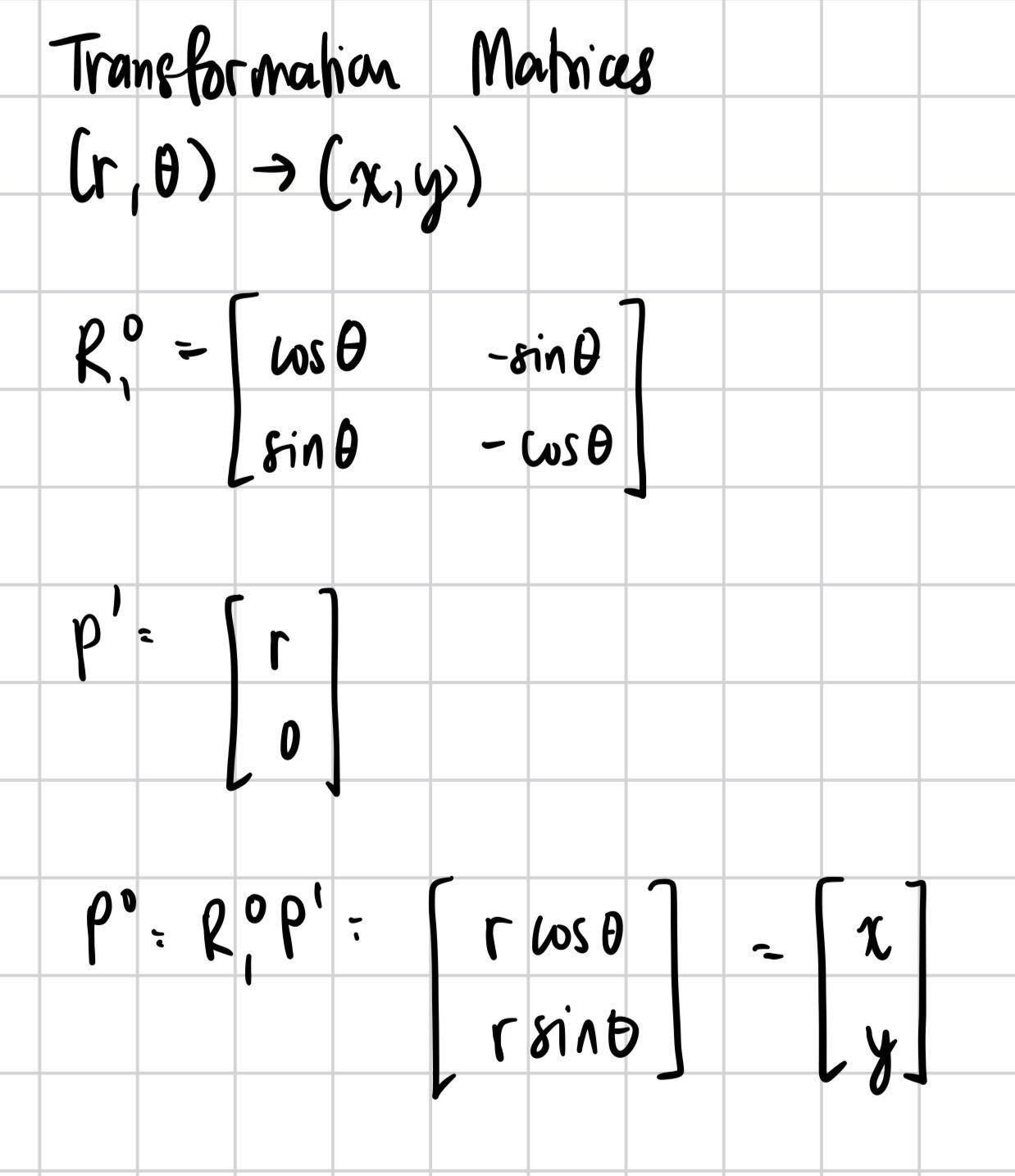
#3 = (5,3) | |
x3 = [] | |
y3 = [] | |
for i in range(0,len(tof1_pt3)): | |
r = tof1_pt3[i] | |
theta = angles_rad_pt3[i] | |
x = (r*np.cos(theta)) + (5*304.8) | |
y = (-r*np.sin(theta)) + (3*304.8) | |
x3.append(x/1000) | |
y3.append(y/1000) | |
plt.scatter(x3, y3) | |
plt.title('(5, 3)') |
 transform.png)
Full Map + Corrections
After transforming all sets of data, I combined them into a single map. From here, I could already start to see the room take shape.
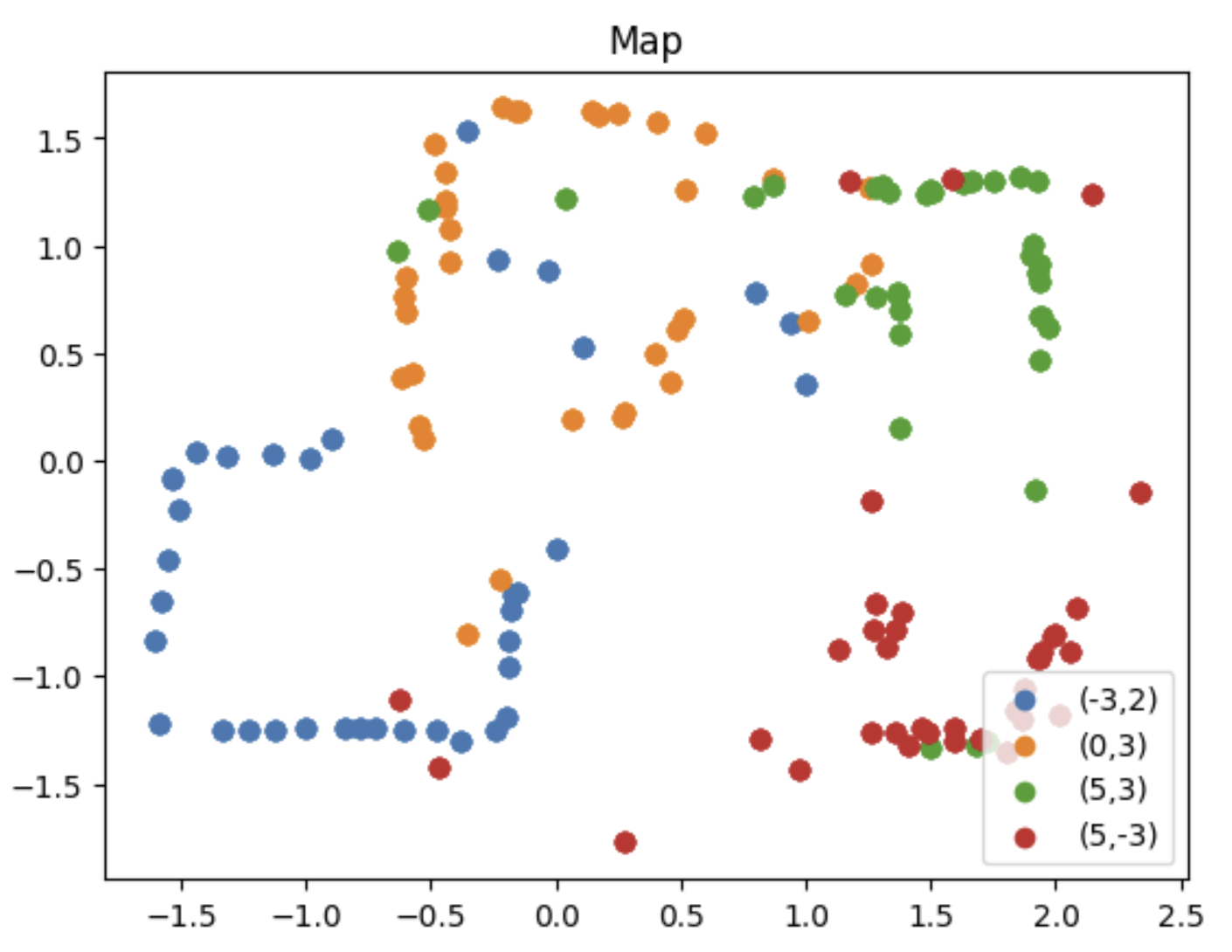
Based on clusters of points, I was able to establish coordinates and draw a box around the perimeter of the area and the obstacles:
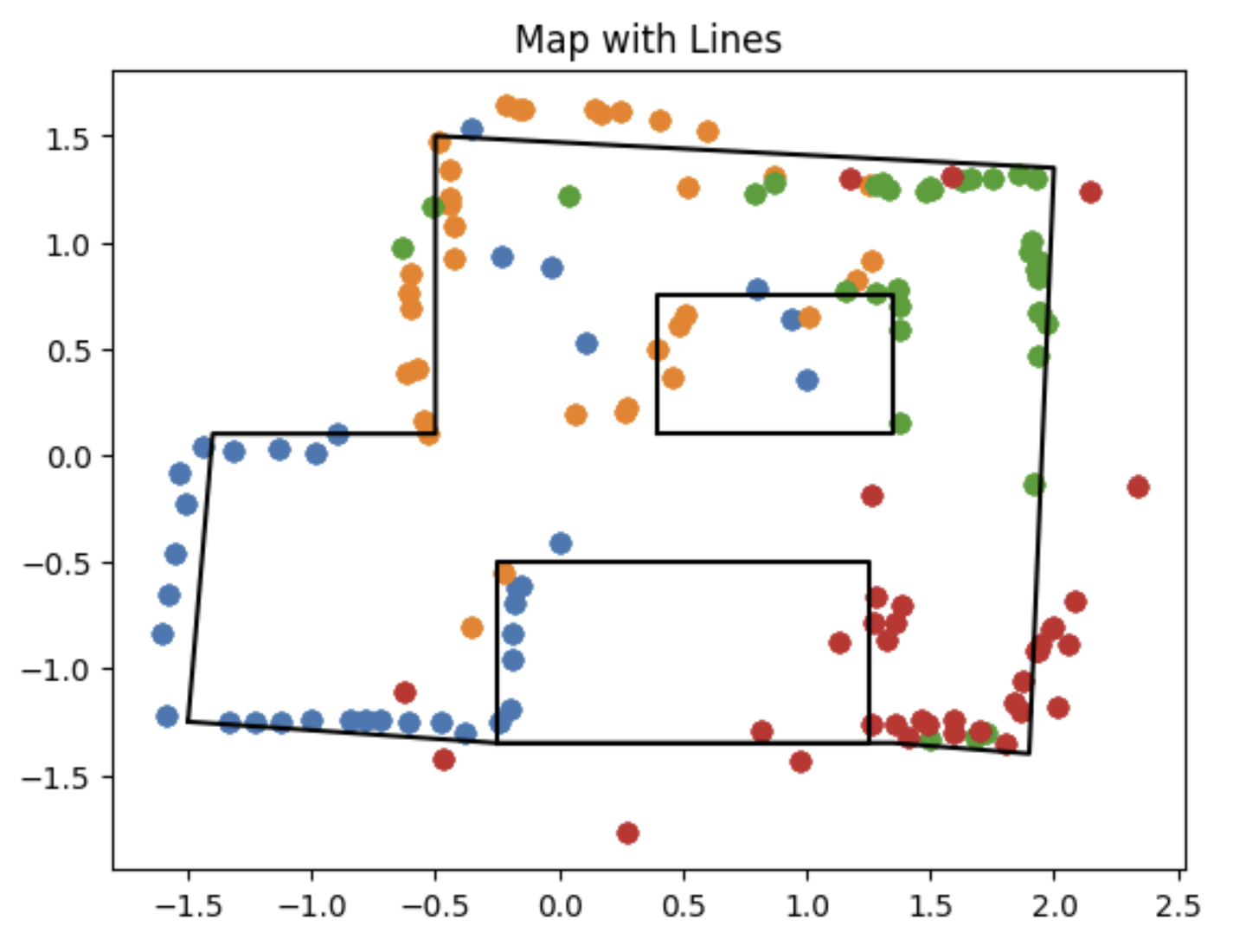
plt.scatter(x1, y1, label = '(-3,2)') | |
plt.scatter(x2, y2, label = '(0,3)') | |
plt.scatter(x3, y3, label = '(5,3)') | |
plt.scatter(x4, y4, label = '(5,-3)') | |
wallx = [-1.5, -1.4, -1.0, -0.5, -0.5, 2.0, 1.9, 1.35, -0.25, -1.5] | |
wally = [-1.25, 0.1, 0.1, 0.1, 1.5, 1.35, -1.4, -1.35, -1.35, -1.25] | |
plt.plot(wallx, wally, color='black') | |
box1x = [0.4,0.4,1.35,1.35,0.4] | |
box1y = [0.1,0.75,0.75,0.1,0.1] | |
plt.plot(box1x, box1y, color='black') | |
box2x = [-0.25, -0.25, 1.25, 1.25, -0.25] | |
box2y = [-1.35, -0.5, -0.5, -1.35, -1.35] | |
plt.plot(box2x, box2y, color='black') | |
plt.title('Map with Lines') |
I noticed two main things about my map:
- It was not perfectly straight, which is most easily seen by the corners (specifically the (-3, -2) data)
- The two boxes in the area were not particularly well detected, specifically by the data take at (5, -3). There is a cluster of red points that could be from the box, but ultimately fall short. This means that in my map, the object appears way larger.
The first issue there can be fixed. I went back and offset the yaw values from each data set until I saw better results. I imagine that this slight variation is due to two things. First, small differences in initial orientation would read to slightly different offsets on the final transformed readings. And secondly, gyro drift may have come into play for the last portion of the rotations, or any slight spinning off axis could mess things up. I ended up offsetting each point by the following angles. Again, (5, -3) was the worst:
- (-3, -2): -6 degrees
- (0, 3): 8 degrees
- (5, 3): 2 degrees
- (5, -3): 14 degrees
Adding these offsets did end up helping the outer perimeter of the map quite a bit, though. Here is the new map:
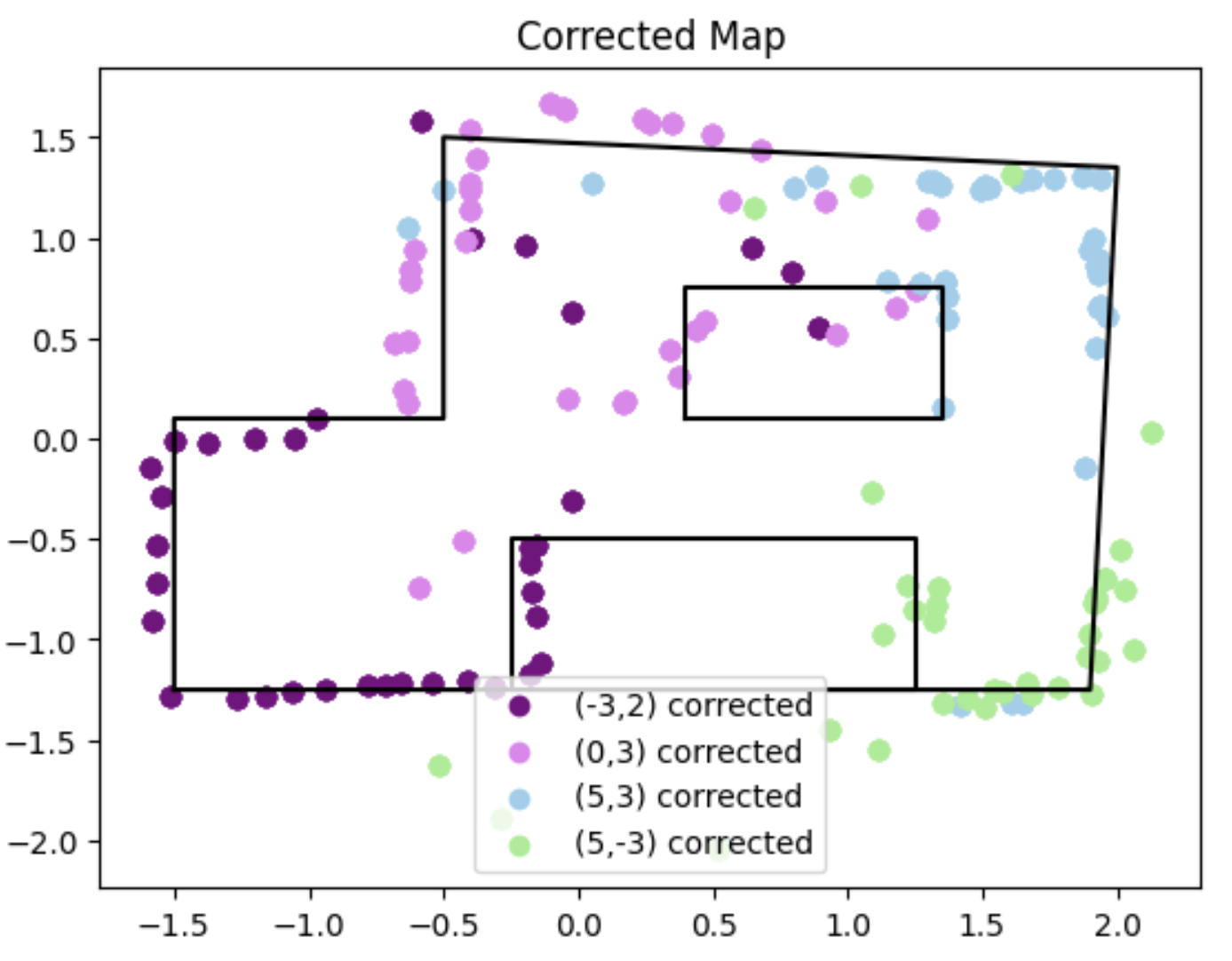
Discussion
This lab showed how sensor data can be used not only to predict robot dynamics, but to understand the local environment. The transformation matrices were very powerful in taking indict data of the room and turning it into a detailed map. I enjoyed this lab!